Fade Camera Background Color Over Time in Unity C# (Free Script)
Here's the code :
using UnityEngine;
public class FadeColor : MonoBehaviour
{
private new Camera camera;
[SerializeField] [Range(0f, 1f)] float lerpTime;
[SerializeField] Color[] myColors;
private int colorIndex = 0;
private float t = 0f;
private int len;
private void Start()
{
camera = GetComponent<Camera>();
len = myColors.Length;
}
private void Update()
{
camera.backgroundColor = Color.Lerp(camera.backgroundColor, myColors[colorIndex], lerpTime * Time.deltaTime);
t = Mathf.Lerp(t, 1f, lerpTime * Time.deltaTime);
if (t > 0.9f)
{
t = 0f;
colorIndex++;
colorIndex = (colorIndex >= len ? 0 : colorIndex);
}
}
}
The Component
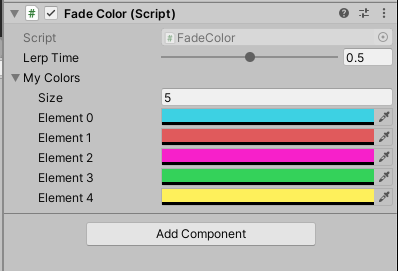
Demo Video on how to use this script coming soon! In the meantime watch a video by : Hamza Herbou that inspired this script.